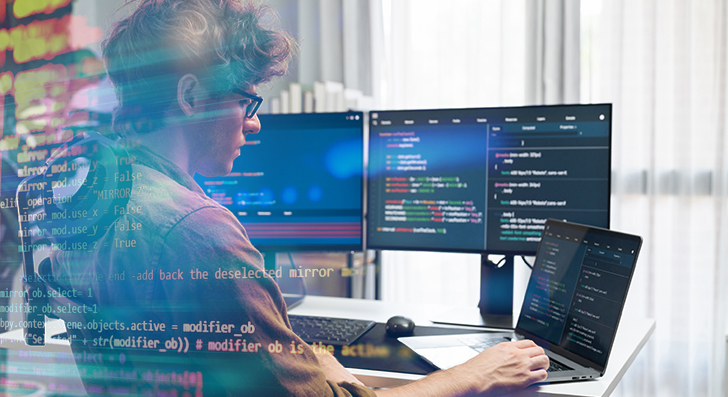
Scalability usually means your application can handle advancement—additional customers, more facts, plus much more site visitors—with out breaking. As a developer, making with scalability in mind will save time and pressure later on. Right here’s a transparent and useful guidebook to assist you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't a thing you bolt on later—it ought to be portion of your system from the beginning. Quite a few programs are unsuccessful if they develop fast due to the fact the original style and design can’t deal with the additional load. As being a developer, you'll want to Feel early about how your process will behave under pressure.
Start off by designing your architecture to get adaptable. Keep away from monolithic codebases where almost everything is tightly connected. As a substitute, use modular design or microservices. These designs break your application into scaled-down, independent areas. Each module or support can scale on its own with no affecting The full system.
Also, contemplate your databases from day one particular. Will it have to have to manage one million customers or maybe a hundred? Select the ideal kind—relational or NoSQL—determined by how your data will expand. Plan for sharding, indexing, and backups early, even if you don’t need to have them nonetheless.
Another essential position is to stop hardcoding assumptions. Don’t write code that only operates underneath recent disorders. Think about what would occur If the user base doubled tomorrow. Would your app crash? Would the database slow down?
Use design and style styles that support scaling, like message queues or function-pushed methods. These support your application handle more requests devoid of finding overloaded.
After you Establish with scalability in your mind, you are not just getting ready for success—you're reducing upcoming problems. A well-prepared process is simpler to keep up, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the correct Database
Selecting the correct database is usually a critical Section of creating scalable applications. Not all databases are built a similar, and using the Incorrect one can slow you down or maybe result in failures as your app grows.
Get started by being familiar with your knowledge. Is it really structured, like rows in the table? If yes, a relational databases like PostgreSQL or MySQL is a good in good shape. These are generally powerful with interactions, transactions, and consistency. In addition they help scaling techniques like browse replicas, indexing, and partitioning to deal with more targeted traffic and information.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your browse and compose patterns. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you handling a weighty generate load? Consider databases that could deal with substantial produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term info streams).
It’s also sensible to Assume in advance. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you gained’t want to change later on.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your information according to your accessibility designs. And constantly keep an eye on databases functionality while you increase.
Briefly, the right databases depends on your application’s composition, velocity desires, And just how you assume it to increase. Just take time to choose properly—it’ll conserve a great deal of difficulties later on.
Optimize Code and Queries
Quick code is essential to scalability. As your application grows, just about every modest delay adds up. Improperly penned code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s essential to Create effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away nearly anything unwanted. Don’t choose the most complex Alternative if an easy 1 is effective. Maintain your functions shorter, targeted, and easy to check. Use profiling instruments to locate bottlenecks—sites the place your code requires much too prolonged to run or works by using a lot of memory.
Future, have a look at your database queries. These typically sluggish things down a lot more than the code itself. Ensure that Every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and instead decide on certain fields. Use indexes to hurry up lookups. And steer clear of undertaking a lot of joins, Specifically throughout large tables.
In case you see the identical facts being requested again and again, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 information may possibly crash if they have to take care of 1 million.
In a nutshell, scalable applications are rapidly applications. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay smooth website and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's to deal with far more end users and a lot more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of a person server executing the many operate, the load balancer routes consumers to various servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When people request the same facts once again—like an item site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two popular different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, increases pace, and makes your app extra productive.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they assist your app take care of more consumers, keep fast, and Recuperate from challenges. If you plan to expand, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you'll need equipment that let your app expand simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to acquire hardware or guess foreseeable future ability. When website traffic improves, you can include a lot more assets with just a couple clicks or routinely employing car-scaling. When website traffic drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and security tools. You are able to concentrate on building your application in lieu of taking care of infrastructure.
Containers are A different essential Device. A container packages your app and all the things it ought to run—code, libraries, settings—into one device. This causes it to be uncomplicated to move your app concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the preferred Device for this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's app crashes, it restarts it quickly.
Containers also help it become easy to different areas of your application into companies. You are able to update or scale pieces independently, that's great for effectiveness and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy easily, and Get well swiftly when complications take place. If you want your app to mature without having restrictions, begin employing these tools early. They preserve time, cut down danger, and make it easier to stay focused on making, not fixing.
Check Anything
In the event you don’t keep an eye on your application, you gained’t know when points go Completely wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a crucial Component of setting up scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it takes for customers to load webpages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for significant challenges. Such as, In the event your reaction time goes earlier mentioned a limit or even a support goes down, you ought to get notified instantly. This assists you fix issues speedy, normally in advance of end users even recognize.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and see a spike in faults or slowdowns, it is possible to roll it back before it will cause true harm.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, checking helps you maintain your app trusted and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you could Develop applications that grow easily without the need of breaking under pressure. Start off little, Consider big, and Construct clever.